Fluid Slider is a slider widget with a popup bubble displaying the precise value selected. The idea was to have a minimal slider design with the value inside the slider that also allows you to see it when you drag the slider hence the value offset animation on tap.
Previously we’ve seen some article on Android Slider like :
Ok, Now lets dive with implementation and coding part and let’s start from Requirement.
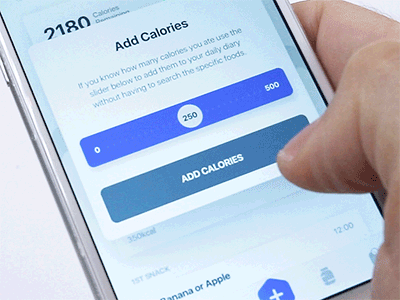
Requirements
- Android 4.1 Jelly Bean (API lvl 16) or greater
- Your favorite IDE
Installation
Just download the package from here and add it to your project classpath, or just use the maven repo:
Gradle:
implementation 'com.ramotion.fluidslider:fluid-slider:0.2.0'
SBT:
libraryDependencies += "com.ramotion.fluidslider" % "fluid-slider" % "0.2.0"
Maven:
<dependency> <groupId>com.ramotion.fluidslider</groupId> <artifactId>fluid-slider</artifactId> <version>0.2.0</version> <type>aar</type> </dependency>
Download Kotlin Fluid Slider :
Add Fluid Slider in layout
- Place the
FluidSlider
in your layout. - To track the current position of the slider, set the
positionListener
, as shown below:
val slider = findViewById<FluidSlider>(R.id.fluidSlider) slider.positionListener = { p -> Log.d("MainActivity", "current position is: $p" )}
- You can also track the beginning and completion of the movement of the slider, using the following properties:
beginTrackingListener
andendTrackingListener
. Example below:
slider.beginTrackingListener = { /* action on slider touched */ } slider.endTrackingListener = { /* action on slider released */ }
Full Source Code
Here is simple example, how to change FluidSlider
range.
// Kotlin val max = 45 val min = 10 val total = max - min val slider = findViewById<FluidSlider>(R.id.fluidSlider) slider.positionListener = { pos -> slider.bubbleText = "${min + (total * pos).toInt()}" } slider.position = 0.3f slider.startText ="$min" slider.endText = "$max" // Java final FluidSlider slider = findViewById(R.id.fluidSlider); slider.setBeginTrackingListener(new Function0<Unit>() { @Override public Unit invoke() { Log.d("D", "setBeginTrackingListener"); return Unit.INSTANCE; } }); slider.setEndTrackingListener(new Function0<Unit>() { @Override public Unit invoke() { Log.d("D", "setEndTrackingListener"); return Unit.INSTANCE; } }); // Or Java 8 lambda slider.setPositionListener(pos -> { final String value = String.valueOf( (int)((1 - pos) * 100) ); slider.setBubbleText(value); return Unit.INSTANCE; });
Some Fluid Slider attributes
See this also : Two Panels Layout Animation Android Library
Here are the attributes you can specify through XML or related setters:
bar_color
– Color of slider.bubble_color
– Color of circle “bubble” inside bar.bar_text_color
– Color ofstart
andend
texts of slider.bubble_text_color
– Color of text inside “bubble”.start_text
– Start (left) text of slider.end_text
– End (right) text of slider.text_size
– Text size.duration
– Duration of “bubble” rise in milliseconds.initial_position
– Initial positon of “bubble” in range form0.0
to1.0
.size
– Height of slider. Can besmall
(40dp) andnormal
(56dp).
Hope you like this article. Please share with your friends.
Share your thoughts