You are going to delete lots of lines of code! Yes, it is not a clickbait! Most Android developers already know that Kotlin Parcelize allows any model class to be in the form of “packages” that will be sent between Android classes as they are developed by the developer.
How do you pass your model between activities?
- Serializable?
- EventBus / Otto ?
- Parcelable?
Serializable = Reflection! Run Forest Run!!! It is terrible for Android performance.
See this : Android Timeline View Library
Bus libraries like EventBus or Otto are easy to use and have good performance but after some time they cause too much complexity in your code. Kotlin Parcelize Parcelable interface is great for Android performance but it has too much boilerplate.
Kotlin Parcelize ?
Kotlin Parcelize added parcelable support in version 1.1.4.
Android Extensions plugin now includes an automatic Parcelable implementation generator. Declare the serialized properties in a primary constructor and add a @Parcelize annotation, and
writeToParcel()/
createFromParcel() methods will be created automatically.
Did you know : How to Create Firefox Extension Using KotlinJS?
Java model vs Kotlin model
I have created two samples of the exact same class with Java and with Kotlin using Parcelable.
Student.java
public class Student implements Parcelable{ private String id; private String name; private String grade; // Constructor public Student(String id, String name, String grade){ this.id = id; this.name = name; this.grade = grade; } // Getter and setter methods ......... ......... // Parcelling part public Student(Parcel in){ String[] data = new String[3]; in.readStringArray(data); // the order needs to be the same as in writeToParcel() method this.id = data[0]; this.name = data[1]; this.grade = data[2]; } @Оverride public int describeContents(){ return 0; } @Override public void writeToParcel(Parcel dest, int flags) { dest.writeStringArray(new String[] {this.id, this.name, this.grade}); } public static final Parcelable.Creator CREATOR = new Parcelable.Creator() { public Student createFromParcel(Parcel in) { return new Student(in); } public Student[] newArray(int size) { return new Student[size]; } }; }
Student.kt ?
@Parcelize data class Student(val id: String, val name: String, val grade: String) : Parcelable
Prerequisites:
Kotlin version 1.1.4 or newer
How To Use:
Add the code below into your gradle.
androidExtensions { experimental = true }
You also need to add apply plugin: ‘kotlin-android-extensions’ to your gradle file. However, if you have created your project using Kotlin support, it should be added automatically.
You must see : Comparison of All Android Dependency Injection
Then add @Parcelize annotation to your model. That’s it! You don’t need to write any parcel methods anymore!
Our awesome Kotlin Parcelize model looks like below:
package com.burakeregar.kotlinparcelize import android.os.Parcelable import kotlinx.android.parcel.Parcelize /** * Created by Burak Eregar on 1.12.2017. * burakeregar@gmail.com * https://github.com/burakeregar */ @Parcelize data class PersonModel(val name: String, val age: Int) : Parcelable
About Lint Error
Android Studio gives the Lint error below right now. It is a reported bug to IntelliJ. You can find the corresponding issue in the link below.
https://youtrack.jetbrains.com/issue/KT-19300
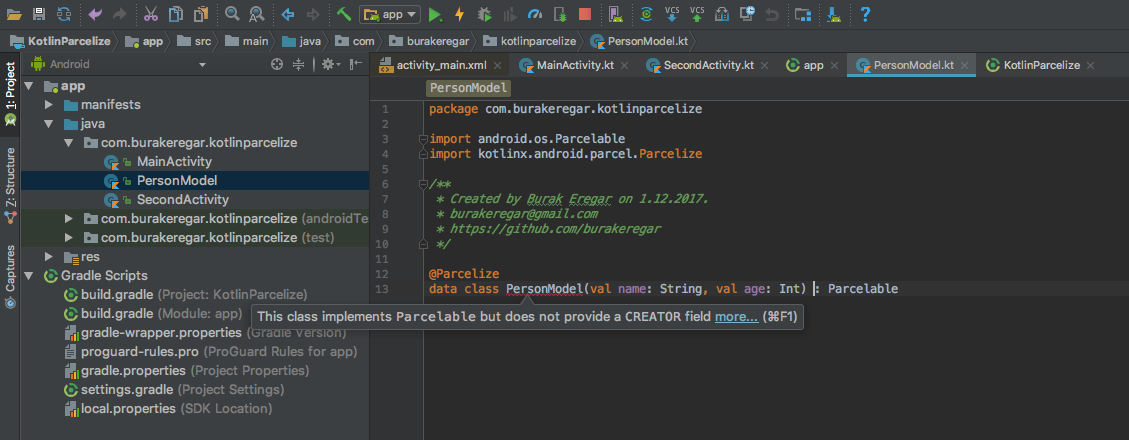
You can run your project without any errors so you can just ignore the Lint error for now.
Please find the sample project for Kotlin Parcelize in the link below.
[button color=”blue” size=”” type=”3d” target=”_blank” link=”https://github.com/burakeregar/KotlinParcelize/archive/master.zip”]Download Kotlin Parcelize Example[/button]
If you liked the article, please clap it. Thanks!
Share your thoughts