Ever wondered how large enterprise scale systems are designed? Before major software development starts, we have to choose a suitable architectural patterns that will provide us with the desired functionality and quality attributes.
Hence, we should understand different architectural patterns, before applying them to our design.
What is an Architectural Pattern?
According to Wikipedia,
An architectural pattern is a general, reusable solution to a commonly occurring problem in software architecture within a given context. Architectural patterns are similar to software design pattern but have a broader scope.
Do you have answer : Will Android support Java 9 after Kotlin updates?
In this article, I will be briefly explaining the following top 10 programming architectural patterns with their usage, pros and cons.
Layered pattern
This pattern is also known as n-tier architecture pattern. It can be used to structure programs that can be decomposed into groups of subtasks, each of which is at a particular level of abstraction. Each layer provides services to the next higher layer.
The most commonly found 4 layers of a general information system are as follows.
- Presentation layer (also known as UI layer)
- Application layer (also known as service layer)
- Business logic layer (also known as domain layer)
- Data access layer (also known as persistence layer)
Usage
- General desktop applications.
- E commerce web applications.
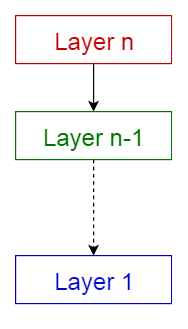
Pros
- A lower layer can be used by different higher layers.
- Layers make standardization easier as we can clearly define levels.
- Changes can be made within the layer without affecting other layers.
Cons
- Not universally applicable.
- Certain layers may have to be skipped in certain situations.
See this : Android Oreo Vs iOS 11 : Comparison you should know about this
Client-server pattern
This pattern consists of two parties; a server and multiple clients. The server component will provide services to multiple client components. Clients request services from the server and the server provides relevant services to those clients. Furthermore, the server continues to listen to client requests.
Usage
- Online applications such as email, document sharing and banking.
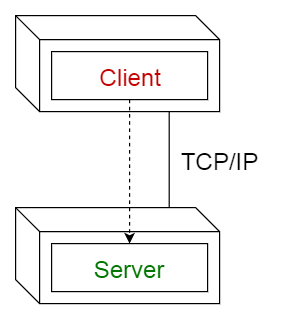
Pros
- Good to model a set of services where clients can request them.
Cons
- Requests are typically handled in separate threads on the server.
- Inter-process communication causes overhead as different clients have different representations.
Recommended : Comparison : Android O Vs Android N
Master-slave pattern
This pattern consists of two parties; master and slaves. The master component distributes the work among identical slave components, and computes a final result from the results which the slaves return.
Usage
- In database replication, the master database is regarded as the authoritative source, and the slave databases are synchronized to it.
- Peripherals connected to a bus in a computer system (master and slave drives).
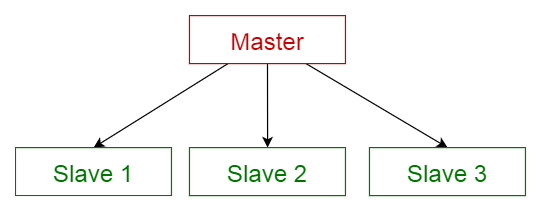
Pros
- Accuracy – The execution of a service is delegated to different slaves, with different implementations.
Cons
- The slaves are isolated: there is no shared state.
- The latency in the master-slave communication can be an issue, for instance in real-time systems.
- This pattern can only be applied to a problem that can be decomposed
Did you know : Problems with Android Oreo – 6 Things you need to know
Best Android Course : Android Course Online 2018
Pipe-filter pattern
This pattern can be used to structure systems which produce and process a stream of data. Each processing step is enclosed within a filter component. Data to be processed is passed through pipes. These pipes can be used for buffering or for synchronization purposes.
Usage
- Compilers. The consecutive filters perform lexical analysis, parsing, semantic analysis, and code generation.
- Workflows in bioinformatics.

Pros
- Exhibits concurrent processing. When input and output consist of streams, and filters start computing when they receive data.
- Easy to add filters. The system can be extended easily.
- Filters are reusable. Can build different pipelines by recombining a given set of filters
Cons
- Efficiency is limited by the slowest filter process.
- Data-transformation overhead when moving from one filter to another.
Broker pattern
This pattern is used to structure distributed systems with decoupled components. These components can interact with each other by remote service invocations. A broker component is responsible for the coordination of communication among components.
Servers publish their capabilities (services and characteristics) to a broker. Clients request a service from the broker, and the broker then redirects the client to a suitable service from its registry.
Usage
- Message broker software such as Apache ActiveMQ, Apache Kafka, RabbitMQ and JBoss Messaging.
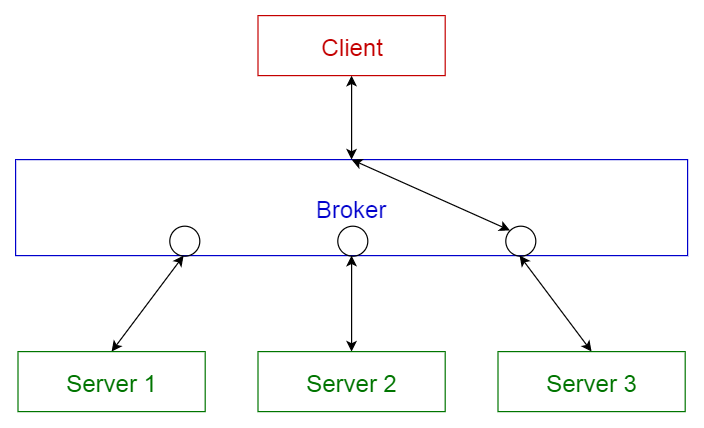
Pros
- Allows dynamic change, addition, deletion and relocation of objects, and it makes distribution transparent to the developer.
Cons
- Requires standardization of service descriptions.
See this also : See What’s New in Android Oreo
Peer-to-peer pattern
In this pattern, individual components are known as peers. Peers may function both as a client, requesting services from other peers, and as a server, providing services to other peers. A peer may act as a client or as a server or as both, and it can change its role dynamically with time.
Usage
- File-sharing networks such as Gnutella and G2)
- Multimedia protocols such as P2PTV and PDTP.
- Proprietary multimedia applications such as Spotify.
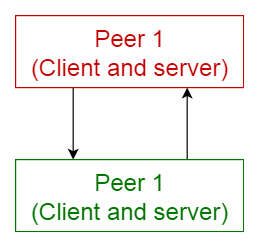
Pros
- Supports decentralized computing.
- Highly robust in the failure of any given node.
- Highly scalable in terms of resources and computing power.
Cons
- There is no guarantee about quality of service, as nodes cooperate voluntarily.
- Security is difficult to be guaranteed.
- Performance depends on the number of nodes.
Event-bus pattern
This pattern is a primarily deals with events and has 4 major components; event source, event listener, channel and event bus. Sources publish messages to particular channels on an event bus. Listeners subscribe to particular channels. Listeners are notified of messages that are published to a channel to which they have subscribed before.
Usage
- Android development
- Notification services
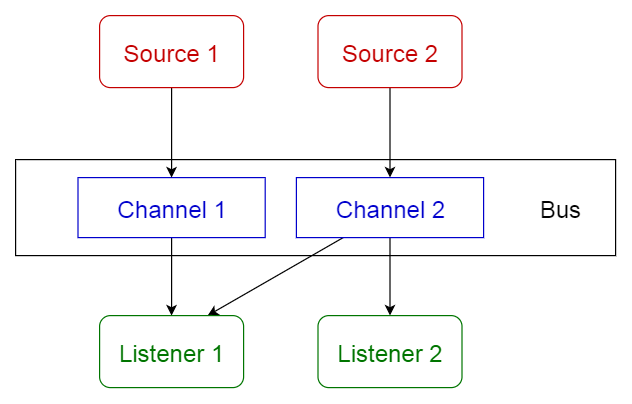
Pros
- New publishers, subscribers and connections can be added easily.
- Effective for highly distributed applications.
Cons
- Scalability may be a problem, as all messages travel through the same event bus
Model-view-controller pattern
This pattern, also known as MVC pattern, divides an interactive application in to 3 parts as,
- model — contains the core functionality and data
- view — displays the information to the user (more than one view may be defined)
- controller — handles the input from the user
This is done to separate internal representations of information from the ways information is presented to, and accepted from, the user. It decouples components and allows efficient code reuse.
Usage
- Architecture for World Wide Web applications in major programming languages.
- Web frameworks such as Django and Rails.
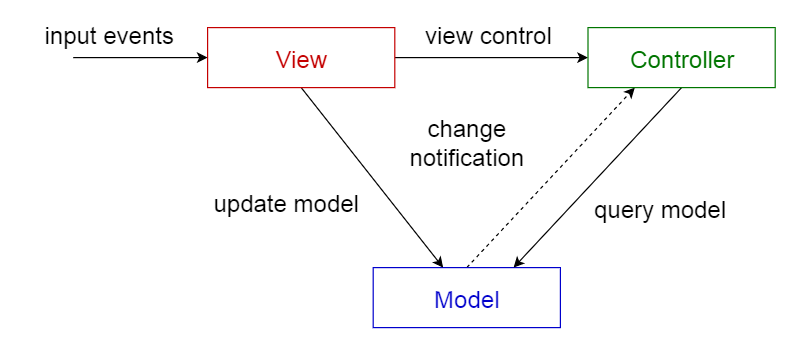
Pros
- Makes it easy to have multiple views of the same model, which can be connected and disconnected at run-time.
Cons
- Increases complexity. May lead to many unnecessary updates for user actions.
Blackboard pattern
This pattern is useful for problems for which no deterministic solution strategies are known. The blackboard pattern consists of 3 main components.
- blackboard — a structured global memory containing objects from the solution space
- knowledge source — specialized modules with their own representation
- control component — selects, configures and executes modules.
All the components have access to the blackboard. Components may produce new data objects that are added to the blackboard. Components look for particular kinds of data on the blackboard, and may find these by pattern matching with the existing knowledge source.
Usage
- Speech recognition
- Vehicle identification and tracking
- Protein structure identification
- Sonar signals interpretation.
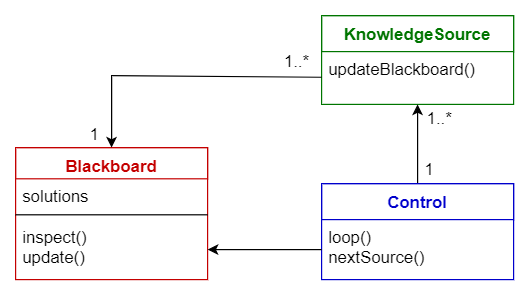
Pros
- Easy to add new applications.
- Extending the structure of the data space is easy.
Cons
- Modifying the structure of the data space is hard, as all applications are affected.
- May need synchronization and access control.
Interpreter pattern
This pattern is used for designing a component that interprets programs written in a dedicated language. It mainly specifies how to evaluate lines of programs, known as sentences or expressions written in a particular language. The basic idea is to have a class for each symbol of the language.
Usage
- Database query languages such as SQL.
- Languages used to describe communication protocols.
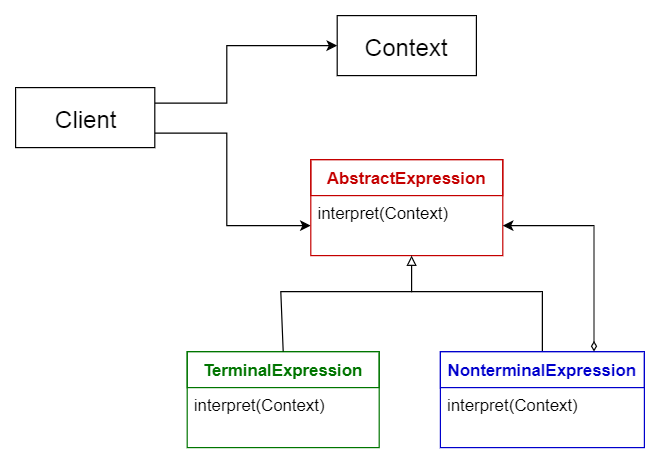
Pros
- Highly dynamic behavior is possible.
- Good for end user programmability.
- Enhances flexibility, because replacing an interpreted program is easy.
Cons
- Because an interpreted language is generally slower than a compiled one, performance may be an issue.
[td_smart_list_end]
Hope you found this article useful. I would love to hear your thoughts. ?
Thanks for reading. ?
Cheers! ?
Share your thoughts